PythonユーザーなのでAnacondaにOpenCVをインストールしました。特に問題なし。
インストール後はチュートリアルを参考にコマンドを覚える作業。
(日本語サイトより公式チュートリアルの方が分かりやすかった。さすが公式。)
歩行者検知

ググッたら二つブログが出てきたので参考にしながら始めました。
”HOG(Histograms of Oriented Gradients)とは局所領域 (セル) の輝度の勾配方向をヒストグラム化したものです。”
”メリットとしては、勾配情報をもとにしているため、異なるサイズの画像を対象とする際も同じサイズにリサイズすることで比較可能になります。”
--画像からHOG特徴量の抽出から引用
...分からない(^ ^;)
HOGの勉強はとりあえず後回しにして実装してしまいましょう!!
実装

OpenCVにはHOG+SVMを用いた人検出器が二種類用意されています。
1. cv2.HOGDescriptor_getDefaultPeopleDetector()
名前の通りデフォルトのこいつは、INRIA Person Datasetという64×128画素のデータセットで学習している検出器で、
2. cv2.HOGDescriptor_getDaimlerPeopleDetector()
こっちはDaimler Pedestrian Detection Benchmark Datasetという48×96画素データで学習している検出器。
二つ目の方が「画像内でサイズの小さい人」も検出できるよ、ということらしいです。
以上の情報を基に、デフォルトを使ったサンプルプログラムも用意されていたので、それをいじりながら書いたノートがこちらです。
(Documentationとそれに関するQ&Aも参考にしました。)
二つの検出器をそれぞれ実装して、パラメータをいじって性能を比べてみました。
実験(パラメータいじり)
「OpenCV 2.4.6でHOGを試してみた」曰く、以下の2パラメータを調整すれば性能が変わるらしいですが。。。
- hit_threshold : 特徴量とSVM分離超平面の間の距離に対する閾値。0が理想。
- group_threshold : 似た検知を減らすための閾値。0だと全くグルーピングされない。
Default
1. hit_threshold=0, group_threshold=0(左), 2(中), 5(右)
2. hit_threshold=1(左), 2(中), 3(右), group_threshold=0
hit_thresholdを上げると、一気に誤検知が減っている気がします。
しかし3まで上げてしまうと手前の男性の検知が消えてしまいました。
この理由はまだよく分かりませんが、hit_thresholdの方が感度が高そうということは分かりました。
Daimler
group_thresholdを上げても奥の女性の検知はできていませんね。
この辺はもっと検知器に関する勉強が必要そうですね。。。
この辺はもっと検知器に関する勉強が必要そうですね。。。
2. hit_threshold=1(左), 2(中), 3(右), group_threshold=0
ラストはDaimlerでhit_thresholdをいじりましたが、想像より良い感じです。
3まで上げた時はかなり正確に検知できています。
Defaultではhit_thresholdが感度高かった気がしたけれど、Daimlerではhit_thresholdの方がフレキシブルに変えられそうなイメージ。。。
おわりに
まだどちらが良いのかはよく分かりませんでした。とりあえずHOGについて勉強します。
動画でやりました→動画で歩行者検知
HOGとかパラメータについてまとめました→歩行者検知でやってること
Deep Learningも試しました→Windows 7(+GPU)でSSD-Keras動かしてみる
HOGとかパラメータについてまとめました→歩行者検知でやってること
Deep Learningも試しました→Windows 7(+GPU)でSSD-Keras動かしてみる
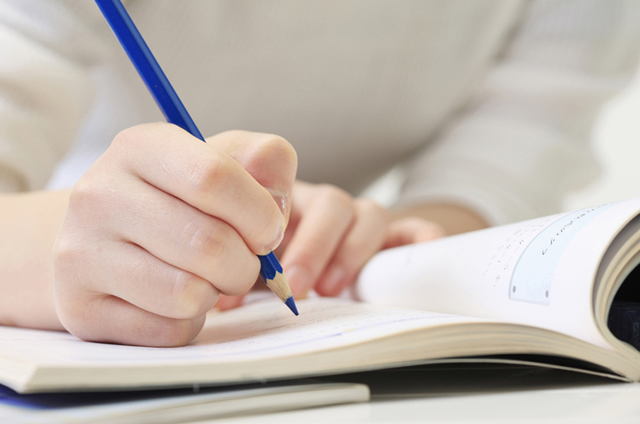
これは動画ファイルでも読み込みは出来るのでしょうか?
返信削除もしよろしければ方法を教えてください。